Back
Invalid `userId` param: Parameter must contain at most 36 chars. Valid chars are a-z, A-Z, 0-9, peri
- 0
- Auth
- Web

Error:
Invalid userId
param: Parameter must contain at most 36 chars. Valid chars are a-z, A-Z, 0-9, period, hyphen, and underscore. Can't start with a special char
This happens when i tried to login, sign up working fine..
this is how i implement the login:
TypeScript
const login = async (email: string, password: string) => {
try {
await account.createSession(email, password)
const user = await account.get()
setCurrentUser(user)
return { email }
} catch (error) {
if (error instanceof Error) {
if (error.message.includes('Invalid credentials')) {
throw new Error('Invalid email or password')
}
}
throw error
}
}
this is for signup
TypeScript
const signup = async (email: string, password: string, name: string) => {
try {
await account.create(ID.unique(), email, password, name)
// After successful signup, log the user in
await login(email, password)
return { name } // Return data for success message
} catch (error) {
if (error instanceof Error) {
if (error.message.includes('already exists')) {
throw new Error(
'An account with this email already exists. Please try logging in instead.',
)
}
// Handle other potential errors
if (error.message.includes('password')) {
throw new Error(
'Password must be at least 8 characters long and contain at least one uppercase letter, one lowercase letter, and one number.',
)
}
if (error.message.includes('email')) {
throw new Error('Please enter a valid email address.')
}
}
throw error
}
}
TL;DR
The error `Invalid 'userId' param: Parameter must contain at most 36 chars` occurs during login due to incorrect user ID format. The valid characters allowed are a-z, A-Z, 0-9, period, hyphen, and underscore, and it can't start with a special character.
For the signup functionality, errors are handled accordingly, such as existing emails, password requirements not met, and invalid email addresses.
Ensure the user ID parameters adhere to the specified format guidelines.
Dependencies:
TypeScript
"dependencies": {
"appwrite": "^17.0.2",
"date-fns": "^4.1.0",
"lucide-react": "^0.509.0",
"next": "15.3.2",
"prettier": "^3.5.3",
"react": "^19.0.0",
"react-dom": "^19.0.0",
"react-hot-toast": "^2.5.2",
"slugify": "^1.6.6",
"zustand": "^5.0.4"
},
Reference: https://appwrite.io/docs/quick-starts/nextjs#step-2
Recommended threads
- Appwrite Fra Cloud Custom Domains Issue
Iām trying to configure my custom domain appwrite.qnarweb.com (CNAME pointing to fra.cloud.appwrite.io with Cloudflare proxy disabled) but encountering a TLS ce...
- Appwrite service :: getCurrentUser :: Us...
Getting this error while creating a react app can someone please help me solve the error
- Storage & Database is not allowing.
Storage & Database is not allowing to CRUD after i have logged in ? Using web SDK with next.js without any SSR or node-sdk.
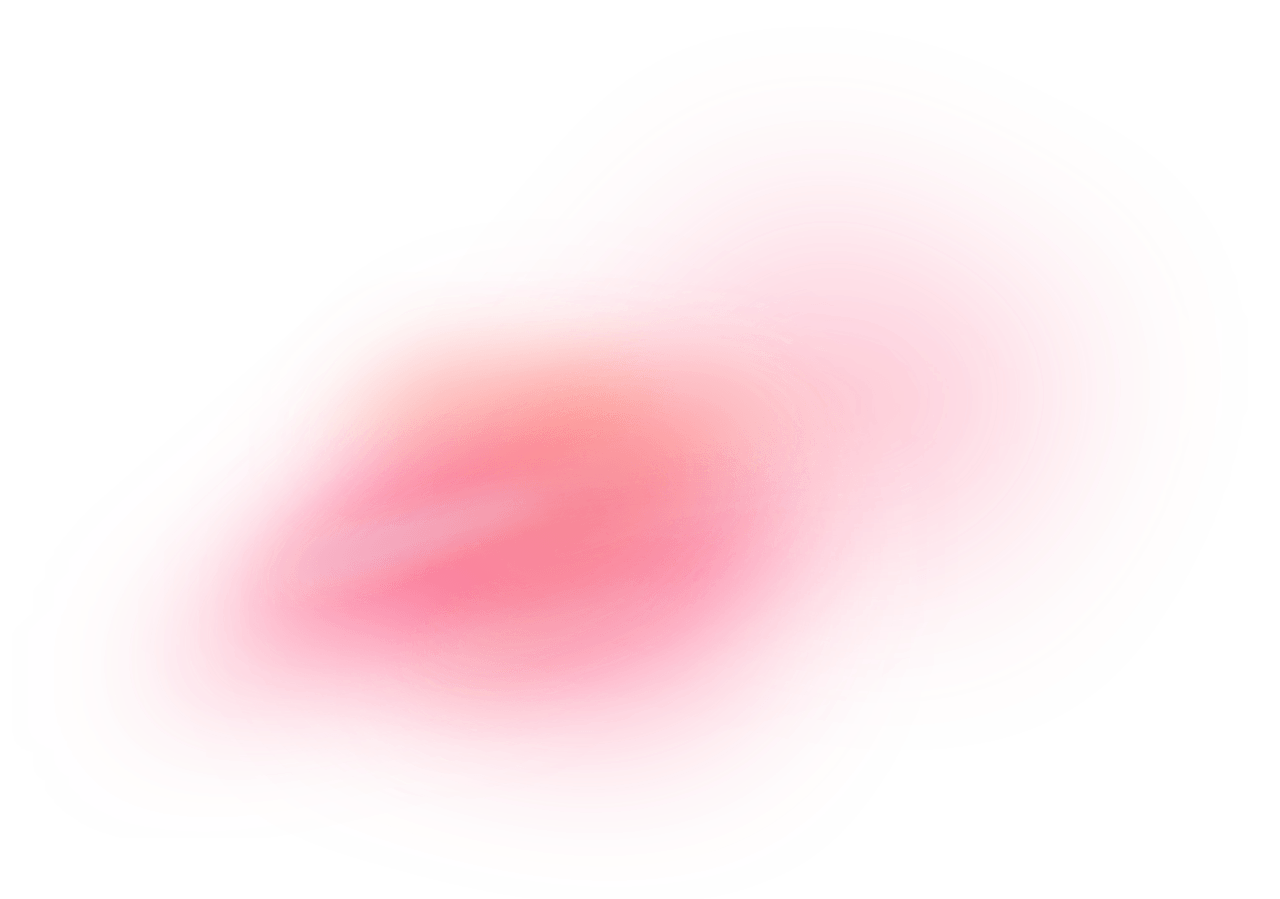